It is a very common requirement where we want to generate reports in PDF, MSWord, MS Excel and HTML format from an OAF page itself without submitting any concurrent program.
To generate the output in PDF or other formats we need the following:
Step 1: Create OAWorkspace, Project, packages, VO and AM (I hope you are already familiar with these)
Step 2: Import following Packages in AM and Controller:
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletResponse;
import oracle.apps.fnd.framework.webui.beans.table.OAAdvancedTableBean;
import oracle.apps.xdo.oa.schema.server.TemplateHelper;
import oracle.jbo.XMLInterface;
Step 3: Create one method in your AM to generate XML output, call this method from your processRequest mathod and get it printed on Jdeveloper console:
Your code for should look like this:
public void getPrintDataXML()
{
try
{
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
OAViewObject vo = (OAViewObject)am.findViewObject(“XXEGASRPrintPageVO1″);
((XMLNode) vo.writeXML(4, XMLInterface.XML_OPT_ALL_ROWS)).print(outputStream);
System.out.println(outputStream.toString());
}
catch(Exception e)
{
throw new OAException (e.getMessage());
}
}
Statements highlighted(BOLD) will print the XML data generated by your view object to Jdeveloper console.
In first line vo.writeXML will generate the XML for VO and print method will write the XML data in outputstream that can further be used to print XML data on Jdeveloper console using println method.

You can copy this output and save with some meaningful name and use it to register as data definition and to design your template(copy only XML data).
Step 4: Design your template
Step 5: Register your Template and data Definition in Oracle Apps

Step 5: Register your Template and data Definition in Oracle Apps
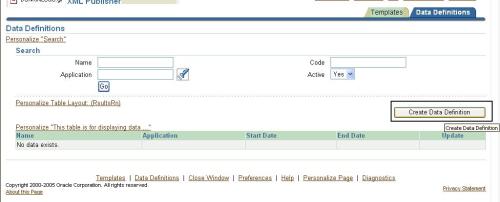
Click on “Create Data Definition” button

Fill in all the mandatory columns and give a short code for your data definition and clink on “Apply” button
now click on “Template” Tab

Click on “Create Template” button

Fill in all the mandatory columns and give a short code (It will be used by XML Publisher APIs to process the template) for your template and clink on “Apply” button
Step 6: Generate output, using XML publisher APIs
Step 6.1: Create a submit button to Generate the output say “Print”
Step 6.2: Add following method to your AM Impl class
public XMLNode getPrintDataXML()//XMLNode
{
OAViewObject vo = (OAViewObject)findViewObject(“EmpVO1″);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
XMLNode xmlNode = (XMLNode) vo.writeXML(4, XMLInterface.XML_OPT_ALL_ROWS);
return xmlNode;
}
Step 6.3: Handle the event for “Print” button:
/*
* Handles functionality of Print button
*/
if(pageContext.getParameter(“btnPrint”)!=null)
{
// Get the HttpServletResponse object from the PageContext. The report output is written to HttpServletResponse.
DataObject sessionDictionary = (DataObject)pageContext.getNamedDataObject(“_SessionParameters”);
HttpServletResponse response = (HttpServletResponse)sessionDictionary.selectValue(null,”HttpServletResponse”);
try {
ServletOutputStream os = response.getOutputStream();
// Set the Output Report File Name and Content Type
String contentDisposition = “attachment;filename=PrintPage.pdf”;
response.setHeader(“Content-Disposition”,contentDisposition);
response.setContentType(“application/pdf”);
// Get the Data XML File as the XMLNode
XMLNode xmlNode = (XMLNode) am.invokeMethod(“getPrintDataXML”);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
ByteArrayInputStream inputStream = new ByteArrayInputStream(outputStream.toByteArray());
ByteArrayOutputStream pdfFile = new ByteArrayOutputStream();
//Generate the PDF Report.
//Process Template
TemplateHelper.processTemplate(
((OADBTransactionImpl)pageContext.getApplicationModule(webBean).getOADBTransaction()).getAppsContext(),
“AK”,//APPLICATION SHORT NAME
“Print Template TMP”, //TEMPLATE_SHORT_CODE
((OADBTransactionImpl)pageContext.getApplicationModule(webBean).getOADBTransaction()).getUserLocale().getLanguage(),
((OADBTransactionImpl)pageContext.getApplicationModule(webBean).getOADBTransaction()).getUserLocale().getCountry(),
inputStream,
TemplateHelper.OUTPUT_TYPE_PDF,
null,
pdfFile);
//TemplateHelper.
// Write the PDF Report to the HttpServletResponse object and flush.
byte[] b = pdfFile.toByteArray();
response.setContentLength(b.length);
os.write(b, 0, b.length);
os.flush();
os.close();
pdfFile.flush();
pdfFile.close();
}
catch(Exception e)
{
response.setContentType(“text/html”);
throw new OAException(e.getMessage(), OAException.ERROR);
}
pageContext.setDocumentRendered(true);
}
Now run your page and click on Print button:

In this manner we can generate the output in Excel, Word and HTML format also but we need to modify our code a bit, few statements in above code are in BOLD…
The statements in BOLD need to be modified:
First file name in following statement :
String contentDisposition = “attachment;filename=PrintPage.pdf”;
In above statement PrintPage.pdf can be replaced with <userdefined_filename>.doc/.xls/.htm
Second is file MIME Type needs to be changed:
response.setContentType(“application/pdf”);
Here application/pdf can be replaced with valid MIME type for Excel/Word and HTML
Third, TemplateHelper.OUTPUT_TYPE_PDF, in this OUTPUT_TYPE_PDF can be replaced by OUTPUT_TYPE_HTML/ OUTPUT_TYPE_EXCEL/ OUTPUT_TYPE_RT
To generate the output in PDF or other formats we need the following:
- XML Data Definition, registered with Apps
- Template, registered with Apps
- XML Publisher APIs to process the template and generate the output in required format.
- To generate XML Data in Jdeveloper Embedded OC4J Sever Log (It will give XML Definition of data; we can use it for designing our Template and register this as Data definition).
- To generate XML data for actual template processing.
Step 1: Create OAWorkspace, Project, packages, VO and AM (I hope you are already familiar with these)
Step 2: Import following Packages in AM and Controller:
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletResponse;
import oracle.apps.fnd.framework.webui.beans.table.OAAdvancedTableBean;
import oracle.apps.xdo.oa.schema.server.TemplateHelper;
import oracle.jbo.XMLInterface;
Step 3: Create one method in your AM to generate XML output, call this method from your processRequest mathod and get it printed on Jdeveloper console:
Your code for should look like this:
public void getPrintDataXML()
{
try
{
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
OAViewObject vo = (OAViewObject)am.findViewObject(“XXEGASRPrintPageVO1″);
((XMLNode) vo.writeXML(4, XMLInterface.XML_OPT_ALL_ROWS)).print(outputStream);
System.out.println(outputStream.toString());
}
catch(Exception e)
{
throw new OAException (e.getMessage());
}
}
Statements highlighted(BOLD) will print the XML data generated by your view object to Jdeveloper console.
In first line vo.writeXML will generate the XML for VO and print method will write the XML data in outputstream that can further be used to print XML data on Jdeveloper console using println method.

You can copy this output and save with some meaningful name and use it to register as data definition and to design your template(copy only XML data).
Step 4: Design your template
Step 5: Register your Template and data Definition in Oracle Apps

Step 5: Register your Template and data Definition in Oracle Apps
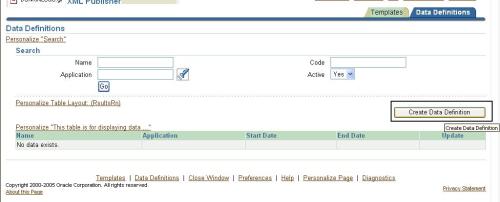
Click on “Create Data Definition” button

Fill in all the mandatory columns and give a short code for your data definition and clink on “Apply” button
now click on “Template” Tab

Click on “Create Template” button

Fill in all the mandatory columns and give a short code (It will be used by XML Publisher APIs to process the template) for your template and clink on “Apply” button
Step 6: Generate output, using XML publisher APIs
Step 6.1: Create a submit button to Generate the output say “Print”
Step 6.2: Add following method to your AM Impl class
public XMLNode getPrintDataXML()//XMLNode
{
OAViewObject vo = (OAViewObject)findViewObject(“EmpVO1″);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
XMLNode xmlNode = (XMLNode) vo.writeXML(4, XMLInterface.XML_OPT_ALL_ROWS);
return xmlNode;
}
Step 6.3: Handle the event for “Print” button:
/*
* Handles functionality of Print button
*/
if(pageContext.getParameter(“btnPrint”)!=null)
{
// Get the HttpServletResponse object from the PageContext. The report output is written to HttpServletResponse.
DataObject sessionDictionary = (DataObject)pageContext.getNamedDataObject(“_SessionParameters”);
HttpServletResponse response = (HttpServletResponse)sessionDictionary.selectValue(null,”HttpServletResponse”);
try {
ServletOutputStream os = response.getOutputStream();
// Set the Output Report File Name and Content Type
String contentDisposition = “attachment;filename=PrintPage.pdf”;
response.setHeader(“Content-Disposition”,contentDisposition);
response.setContentType(“application/pdf”);
// Get the Data XML File as the XMLNode
XMLNode xmlNode = (XMLNode) am.invokeMethod(“getPrintDataXML”);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
ByteArrayInputStream inputStream = new ByteArrayInputStream(outputStream.toByteArray());
ByteArrayOutputStream pdfFile = new ByteArrayOutputStream();
//Generate the PDF Report.
//Process Template
TemplateHelper.processTemplate(
((OADBTransactionImpl)pageContext.getApplicationModule(webBean).getOADBTransaction()).getAppsContext(),
“AK”,//APPLICATION SHORT NAME
“Print Template TMP”, //TEMPLATE_SHORT_CODE
((OADBTransactionImpl)pageContext.getApplicationModule(webBean).getOADBTransaction()).getUserLocale().getLanguage(),
((OADBTransactionImpl)pageContext.getApplicationModule(webBean).getOADBTransaction()).getUserLocale().getCountry(),
inputStream,
TemplateHelper.OUTPUT_TYPE_PDF,
null,
pdfFile);
//TemplateHelper.
// Write the PDF Report to the HttpServletResponse object and flush.
byte[] b = pdfFile.toByteArray();
response.setContentLength(b.length);
os.write(b, 0, b.length);
os.flush();
os.close();
pdfFile.flush();
pdfFile.close();
}
catch(Exception e)
{
response.setContentType(“text/html”);
throw new OAException(e.getMessage(), OAException.ERROR);
}
pageContext.setDocumentRendered(true);
}
Now run your page and click on Print button:

In this manner we can generate the output in Excel, Word and HTML format also but we need to modify our code a bit, few statements in above code are in BOLD…
The statements in BOLD need to be modified:
First file name in following statement :
String contentDisposition = “attachment;filename=PrintPage.pdf”;
In above statement PrintPage.pdf can be replaced with <userdefined_filename>.doc/.xls/.htm
Second is file MIME Type needs to be changed:
response.setContentType(“application/pdf”);
Here application/pdf can be replaced with valid MIME type for Excel/Word and HTML
Third, TemplateHelper.OUTPUT_TYPE_PDF, in this OUTPUT_TYPE_PDF can be replaced by OUTPUT_TYPE_HTML/ OUTPUT_TYPE_EXCEL/ OUTPUT_TYPE_RT
No comments:
Post a Comment